티스토리 뷰
반응형
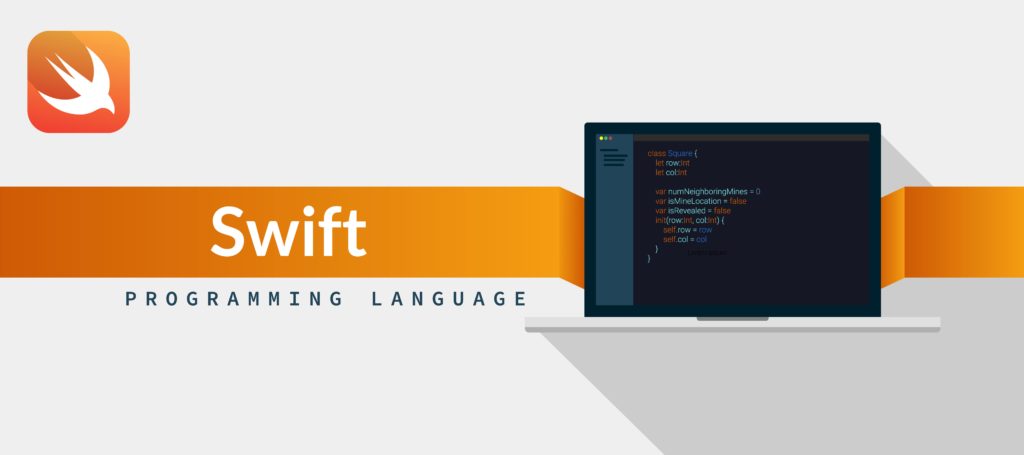
프로퍼티(Property)
Property의 정의
- 클래스나 구조체, 열거체의 객체 인스턴스가 그 내부에 가지고 있는 객체의 상태에 관한 정보를 말합니다(Properties associate values with a particular class, structure, or enumeration).
- 저장 프로퍼티는 상수나 변수를 인스턴스의 일부로서 저장하지만, 연산 프로퍼티는 저장한다기보다 값을 연산하는 프로퍼티입니다(Stored properties store constant and variable values as part of an instance, whereas computed properties calculate (rather than store) a value).
- 저장 프로퍼티는 클래스와 구조체에만 있지만 연산 프로퍼티는 클래스, 구조체 뿐만 아니라 열거체에도 존재할 수 있습니다(Computed properties are provided by classes, structures, and enumerations. Stored properties are provided only by classes and structures).
저장 프로퍼티(Store Properties)
- 저장 프로퍼티는 단순히 값을 저장하고 있는 프로퍼티입니다. 이 프로퍼티는 let이나 var를 이용해서 선언 가능합니다.
- 구조체나 클래스는 저장프로퍼티를 가질 수 있지만 열거형은 갖지 못합니다.
- 구조체를 상수로 선언하면 그 구조체 인스턴스의 프로퍼티는 변경 불가능합니다.
-
struct Car {var modelName: Stringvar speed: Int = 0init(modelName: String) {self.modelName = modelName}}let benz: Car = Car(modelName: "S class")benz.speed = 10 // 오류 발생
cs - 하지만 클래스를 인스턴스로 선언하면 그 프로퍼티는 변경 가능합니다. 클래스는 참조타입이기 때문에 주소를 참조하고 있으며 값을 변경하면 메모리의 다른 값을 바라보기 때문입니다.
-
class Car {var modelName: Stringvar speed: Int = 0init(modelName: String) {self.modelName = modelName}}let benz: Car = Car(modelName: "S class")benz.speed = 10
cs
연산 프로퍼티(Computed Properties)
- 저장 프로퍼티와 다르게 클래스, 구조체, 열거형 모두 연산 프로퍼티를 가질 수 있습니다.
- 연산 프로퍼티는 실제 값을 저장하는 것이 아니라 getter와 setter를 제공해 값을 탐색하고 간접적으로 다른 프로퍼티 값을 설정할 수 있는 방법을 제공합니다.
-
struct Point {var x = 0.0, y = 0.0}struct Size {var width = 0.0, height = 0.0}struct Rect {var origin = Point()var size = Size()var center: Point {get {let centerX = origin.x + (size.width / 2)let centerY = origin.y + (size.height / 2)return Point(x: centerX, y: centerY)}set(newCenter) {origin.x = newCenter.x - (size.width / 2)origin.y = newCenter.y - (size.height / 2)}}}var square = Rect(origin: Point(x: 0.0, y: 0.0),size: Size(width: 10.0, height: 10.0))let initialSquareCenter = square.centersquare.center = Point(x: 15.0, y: 15.0)print("square.origin is now at (\(square.origin.x), \(square.origin.y))")// "square.origin is now at (10.0, 10.0)" 출력
cs - 읽기전용 연산 프로퍼티(Read-Only Computed Properties)
- getter만 있고 setter를 제공하지 않는 연산 프로퍼티를 읽기전용 연산 프로퍼티라고 하며, 반드시 반환값을 제공하며 다른 값은지정할 수 없습니다.
연산 프로퍼티는 반드시 var로 선언해야합니다. 보통 읽기전용은 한번 값이 정해지면 변하지 않기 때문에 상수(let) 선언 해야하지만 연산 프로퍼티는 계산된 값에 따라 값이 변할 수 있기 때문에 var로 선언해야합니다.
프로퍼티 옵저버(Property Observers)
- 프로퍼티에는 새 값이 설정(set) 될 때마다 이벤트를 감지할 수 있는 옵저버를 제공합니다. 프로퍼티 옵저버는 새 값이 이전 값과 같더라도(변경이 발생하지 않아도) 항상 호출됩니다. 프로퍼티에는 다음 두가지 옵저버를 제공합니다.
-
class StepCounter {var totalSteps: Int = 0 {willSet(newTotalSteps) {print("About to set totalSteps to \(newTotalSteps)")}didSet {if totalSteps > oldValue {print("Added \(totalSteps - oldValue) steps")}}}}let stepCounter = StepCounter()stepCounter.totalSteps = 200// About to set totalSteps to 200// Added 200 stepsstepCounter.totalSteps = 360// About to set totalSteps to 360// Added 160 stepsstepCounter.totalSteps = 896// About to set totalSteps to 896// Added 536 steps
cs
- willSet: 값이 저장되기 바로 직전에 호출됩니다. 새 값의 parameter명을 지정할 수 있는데 지정하지 않을 경우 default로 newValue가 사용됩니다.
- didSet: 새 값이 저장되고 난 직후에 호출됩니다. 바뀌기 전의 parameter명을 지정할 수 있는데, 지정하지 않으면 기본 값으로 oldValue를 사용합니다.
타입 프로퍼티(Type Properties)
- 인스턴스 프로퍼티는 생성된 특정 인스턴스에 속한 프로퍼티입니다. 이 프로퍼티는 인스턴스에 종속되어 인스턴스가 생성될 때마다 각 인스턴스에 독립적으로 존재합니다. 반면 타입프로퍼티는 그 타입 자체에 종속되어 해당 타입에서 생성된 모든 인스턴스에서 공유하는 값입니다. 즉, 특정 타입의 모든 인스턴스에서 공통으로 사용되는 값을 정의할 때 유용합니다.
- Note:
- 인스턴스 프로퍼티의 경우 초기값 지정없이 생성하여 인스턴스를 생성할 때 프로퍼티의 값을 받을 수 있습니다. 하지만 타입 프로퍼티의 경우 Initializer가 없어 초기화 할 곳이 없기 때문에 항상 초기값을 지정해서 사용해야 합니다.
- How to use: 열거형과 구조체에서는 타입 프로퍼티는 static 키워드만을 사용합니다. 열거형, 구조체와는 다르게 클래스에서는 static 과 class 두가지 형태의 타입 프로퍼티를 선언할 수 있습니다.
- class로 선언된 프로퍼티는 서브클래스에서 오버라이드 가능합니다.
-
struct SomeStructure {static var storedTypeProperty = "Some value."static var computedTypeProperty: Int {return 1}}enum SomeEnumeration {static var storedTypeProperty = "Some value."static var computedTypeProperty: Int {return 6}}class SomeClass {static var storedTypeProperty = "Some value."static var computedTypeProperty: Int {return 27}class var overrideableComputedTypeProperty: Int {return 107}}
cs
reference: https://docs.swift.org/swift-book/LanguageGuide/Properties.html
반응형
'iOS 앱개발 > Swift Basic' 카테고리의 다른 글
[Swift] - 튜플(Tuples), Range (0) | 2021.03.30 |
---|---|
[Swift ] - Protocol(프로토콜) vs Inheritance(상속) (0) | 2021.03.30 |
[Swift] 열거형(Enum) (0) | 2021.03.23 |
[Swift] Datatype (0) | 2021.03.23 |
[Swift] if let vs guard let (0) | 2021.03.23 |
댓글
반응형
공지사항
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday
링크
TAG
- 공유기 설치#BOJ#이분탐색#Python
- Swift#Tuples#Range
- 백준 알고리즘#BackTracking
- 랜선자르기#이분탐색#BOJ#Python
- 병든 나이트#BOJ#탐욕법#Python
- 순열사이클#BOJ#Python
- django#slicing
- 미로 탐색#백준알고리즘#Python
- 반복수열#백준알고리즘#Python
- 종이자르기#분할정복#BOJ#Python
- 리모컨#완전탐색#BOJ#Python
- Brackets#Stacks and Queues#Codility#Python
- API#lazy#
- 텀 프로젝트#백준알고리즘#Python
- 파이썬알고리즘인터뷰#4장
- django
- Triangle#Sorting#Codility#Python
- 암호코드#dp#BOJ#Python
- 나무자르기#BOJ#이분탐색#Python
- N으로 표현#DP#Programmers#Python
- filter#isalnum#lower
- 쿼드트리#BOJ#분할정복#Python
- 터틀비치#리콘#xbox#controller
- PassingCars#Codility#Python
- Distinct#Codility#Python
- NumberofDiscIntersections#Codility#Sort#Python
- 배열합치기#분할정복#BOJ#Python
- 섬의개수#백준알고리즘#Python
- 토마토#백준알고리즘#Python
- 날짜 계산#BOJ#완전탐색#Python
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
글 보관함